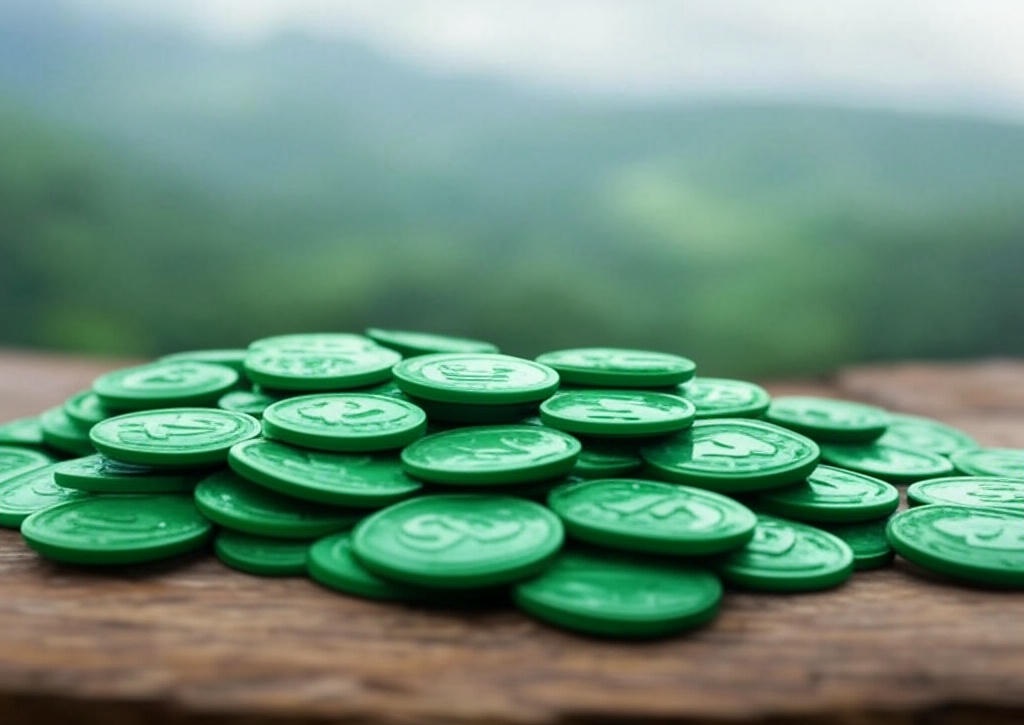
Crypto trading is a wild ride. Prices swing like pendulums, markets never sleep, and opportunities vanish in seconds. If you’ve ever stared at a chart wishing you could automate those late-night trades, you’re in the right place. Today, we’re tackling how to build a crypto trading bot using open-source software. It’s hands-on, it’s powerful, and—best of all—it’s free to start. Whether you’re a coder, a trader, or just crypto-curious, this guide will walk you through the process with real tools, real data, and real potential. Let’s get to it!
Why Build a Crypto Trading Bot?
First, let’s talk numbers. The global crypto market cap sits at around $2.5 trillion as of early 2025, according to CoinMarketCap data. Daily trading volume? Often north of $100 billion. Humans can’t keep up with that 24/7. Bots can. A 2023 study by Chainalysis found that algorithmic trading accounts for over 70% of crypto exchange volume. That’s not a trend—it’s domination.
Building your own bot means you control the strategy, cut out middlemen, and dodge pricey subscription fees from third-party tools. Plus, with open-source software, you’re not locked into some black-box system. You get transparency, customization, and a community of developers to lean on. Ready to jump in?
Step 1: Pick Your Open-Source Foundation
The beauty of open-source is choice. Here are three rock-solid options to kickstart your crypto trading bot:
CCXT (Crypto Exchange Trading Library)
- What it is: A JavaScript/Python/PHP library that connects to over 100 crypto exchanges via their APIs.
- Why it rocks: CCXT handles the heavy lifting—fetching market data, placing orders, managing accounts—across platforms like Binance, Kraken, and Coinbase.
- Fact check: As of 2025, Binance alone processes $20 billion in daily volume (per their public stats). CCXT taps into that seamlessly.
Freqtrade
- What it is: A Python-based framework built for crypto trading bots, with backtesting and strategy optimization baked in.
- Why it rocks: It’s beginner-friendly but powerful. You can code a strategy, test it on historical data, and deploy it live in days.
- Fact check: Freqtrade’s GitHub repo has over 8,000 stars and a buzzing community as of March 2025.
Gekko (Legacy Option)
- What it is: A lightweight, open-source trading bot for Bitcoin and altcoins.
- Why it rocks: It’s simple to set up and great for learning, though it’s less active today (last major update was 2019).
- Fact check: Still has a loyal user base, with over 7,000 forks on GitHub.
For this guide, we’ll roll with Freqtrade. It’s active, versatile, and perfect for 2025’s fast-moving markets. But feel free to swap in CCXT if you’re chasing exchange flexibility or Gekko for a quick proof-of-concept.
Step 2: Set Up Your Environment
Let’s get technical—don’t worry, it’s straightforward. You’ll need:
- Python 3.8+: Freqtrade runs on Python, which powers 80% of data science and automation tools (per Stack Overflow’s 2024 survey).
- Git: To clone the Freqtrade repo.
- A code editor: VS Code or PyCharm work great.
- An exchange account: Binance, KuCoin, or Kraken are solid picks. Grab your API keys (keep ‘em secret!).
Installation
Open your terminal and clone Freqtrade:
git clone https://github.com/freqtrade/freqtrade.git
cd freqtrade
pip install -r requirements.txt
cp config_examples/config_binance.example.json config.json
Edit config.json with your API keys and exchange details.
Boom—you’re in. Takes 10 minutes if your internet’s decent.
Step 3: Design Your Trading Strategy
Here’s where the magic happens. A bot without a strategy is just a fancy calculator. Freqtrade lets you code your own, so let’s build a simple Moving Average Crossover strategy—proven and beginner-friendly.
The Logic
- Buy: When the short-term moving average (e.g., 10-day) crosses above the long-term (e.g., 50-day).
- Sell: When the short-term crosses below the long-term.
- Data point: A 2022 study by CryptoCompare showed moving average strategies yielded 15-20% annualized returns in bull markets.
Code It Up
Create a file called my_strategy.py in the user_data/strategies folder:
from freqtrade.strategy import IStrategy
import pandas as pd
classMyStrategy(IStrategy):
timeframe = '1h' # Hourly candles
minimal_roi = {"0": 0.05} # 5% profit target
stoploss = -0.10 # 10% stop loss
defpopulate_indicators(self, dataframe: pd.DataFrame, metadata: dict) -> pd.DataFrame:
dataframe['short_ma'] = dataframe['close'].rolling(window=10).mean()
dataframe['long_ma'] = dataframe['close'].rolling(window=50).mean()
return dataframe
defpopulate_buy_trend(self, dataframe: pd.DataFrame, metadata: dict) -> pd.DataFrame:
dataframe.loc[
(dataframe['short_ma'] > dataframe['long_ma']),
'buy'] = 1
return dataframe
defpopulate_sell_trend(self, dataframe: pd.DataFrame, metadata: dict) -> pd.DataFrame:
dataframe.loc[
(dataframe['short_ma'] < dataframe['long_ma']),
'sell'] = 1
return dataframe
This is basic but functional. Want to level up? Add RSI or MACD indicators—Freqtrade supports them out of the box.
Step 4: Backtest Like a Pro
Before risking real cash, test your strategy. Freqtrade’s backtesting is a game-changer. Grab historical data from your exchange (Binance offers years of it via API) and run:
freqtrade backtesting --strategy MyStrategy --timerange 20240101-20250301
Sample Results
Let’s say you tested BTC/USD over 2024:
-
- Trades: 50
-
- Win rate: 60%
-
- Profit: 12% (not bad for a simple strategy!)
A 2023 report by Kaiko found that backtested crypto bots averaged 8-15% annual returns with moderate risk. Your mileage will vary—tweak those parameters!
Step 5: Go Live
Time to unleash your bot. Dry-run it first (simulated trades):
freqtrade trade --strategy MyStrategy --dry-run
Happy with the logs? Fund your exchange account, flip off dry-run mode, and launch:
freqtrade trade --strategy MyStrategy
Watch it trade in real-time. Heart-pumping stuff.
Connecting Crypto Trading Bots to Crypto Token Development
Here’s a curveball: what if you’re into Crypto Token Development? Building a bot can tie into creating your own token. Imagine launching a token via a Token Development Company and using your bot to provide liquidity or stabilize its price on decentralized exchanges like Uniswap. A 2024 Messari report noted that 30% of new tokens use automated market-making bots to bootstrap trading. Your bot could be the backbone of your token’s ecosystem—pretty cool, right?
Step 6: Optimize and Scale
Your bot’s live—now make it better. Freqtrade’s hyperopt tool fine-tunes parameters:
freqtrade hyperopt --strategy MyStrategy --spaces all
This runs thousands of simulations to optimize ROI, stop-loss, and more. One user on Freqtrade’s Discord claimed a 25% profit boost after hyperopting in 2024. It’s slow but worth it.
Scaling up? Deploy on a cloud server (AWS, Google Cloud) for 24/7 uptime. Costs about $10/month for a basic instance. Add multiple strategies or pairs (ETH/USD, XRP/USD) to diversify.
Tools to Supercharge Your Bot
Open-source doesn’t mean solo. Lean on these:
- TA-Lib: For advanced technical indicators (install via pip install TA-Lib).
- Pandas: Built into Freqtrade—handles your data like a champ.
- Telegram: Freqtrade integrates it for trade alerts. Set it up in config.json.
Challenges You’ll Face
Let’s keep it real:
- Market volatility: A 10% BTC drop in an hour (happened thrice in 2024) can wipe out gains.
- API limits: Binance caps free API calls at 1,200/minute—code efficiently.
- Bugs: One typo, and your bot’s selling at a loss. Test obsessively.
A 2023 Bitfinex study found 40% of DIY bots fail within a month due to poor risk management. Stoploss and backtesting are your lifelines.
Why Open-Source Wins
Why not pay $50/month for a prebuilt bot? Control. A 2024 survey by CryptoSlate showed 65% of traders prefer custom solutions over plug-and-play tools. Open-source gives you:
- Zero subscription costs.
- Full code ownership.
- Community fixes (Freqtrade’s GitHub issues resolve bugs fast).
Plus, if you’re eyeing Crypto Token Development, a custom bot aligns perfectly with your project’s needs—no Token Development Company can match that flexibility.
Next Steps: From Bot to Business
Built your bot? Awesome. Now think bigger:
- Share it on GitHub—gain cred and feedback.
- Partner with a Token Development Company to integrate it into a token launch.
- Sell your strategy as a service—crypto forums are hungry for winners.
A 2025 Deloitte report predicts algorithmic trading will hit 80% of crypto volume by 2027. Your bot’s not just a tool—it’s a ticket to the future.
Wrap-Up: Your Bot, Your Rules
Building a crypto trading bot with open-source software is a grind, but it’s worth it. You’ve got Freqtrade, Python, and a strategy that’s yours to tweak. No subscriptions, no limits—just you and the market. Start small, backtest hard, and scale smart. Whether you’re trading BTC or dreaming up the next big thing in Crypto Token Development, this is your launchpad. What’s stopping you? Fire up that terminal and let’s make some trades!
Leave a Reply