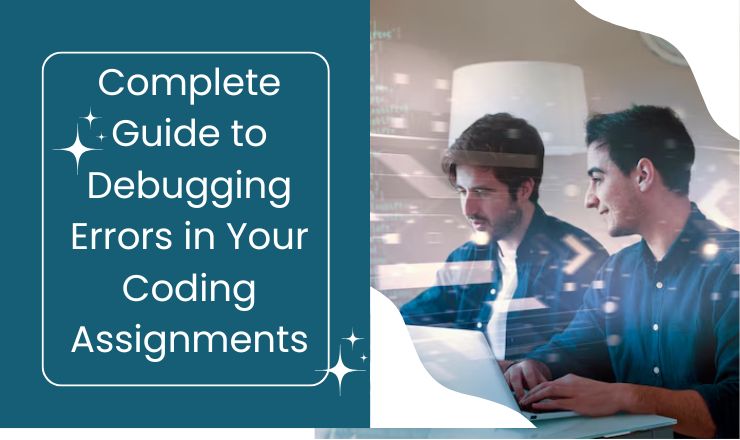
Debugging is an essential skill for any programmer, especially when working on coding assignments. Whether you are a beginner or an advanced developer, encountering errors in your code is inevitable. This guide will provide you with effective strategies to identify, analyze, and fix errors in your coding assignments efficiently. If you are looking for expert assistance, Coding Assignment Help services can provide valuable insights and solutions.
Understanding the Importance of Debugging
Debugging is the process of identifying and resolving errors in a program to ensure it functions correctly. It is crucial because errors can lead to incorrect outputs, system crashes, and inefficient code. By learning effective debugging techniques, you can enhance your programming skills and reduce frustration when working on coding assignments.
Common Types of Errors in Coding Assignments
Before diving into debugging techniques, it’s important to understand the common types of errors that occur in programming:
-
Syntax Errors: These happen when the code deviates from the grammar of the language. Examples include missing semicolons, incorrect indentation, or using undeclared variables.
-
Logical Errors: These are harder to detect as the program runs without crashing but produces incorrect results due to flawed logic.
-
Runtime Errors: These occur during program execution and may result from operations like division by zero, accessing invalid memory locations, or using uninitialized variables.
-
Compilation Errors: These errors prevent the code from being compiled into an executable program due to syntax or semantic issues.
-
Semantic Errors: These occur when the meaning of the code is incorrect, even if the syntax is correct, leading to unintended behavior.
If you are struggling with such errors, opting for programming assignment help can ensure that your code runs efficiently and correctly.
Effective Debugging Techniques
1. Read Error Messages Carefully
Most modern programming languages provide detailed error messages that indicate what went wrong and where. Read these messages carefully to identify the root cause of the error.
2. Use Print Statements for Debugging
One of the simplest debugging methods is using print statements to check variable values and program flow. This helps identify where the code is deviating from expected behavior.
3. Utilize Debugging Tools
Many Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Eclipse provide built-in debugging tools. Features such as breakpoints, step-through execution, and variable inspection can help analyze code execution line by line.
4. Check for Typos and Syntax Issues
Sometimes, a small typo or syntax mistake can cause major errors. Double-check your code for misplaced brackets, missing colons, or incorrect function names.
5. Break Down the Code into Smaller Parts
Instead of debugging an entire program at once, break it down into smaller segments and test each part separately. This facilitates identifying the error’s location.
6. Use Online Debugging Resources
Platforms like Stack Overflow, GitHub discussions, and official documentation can provide insights into common debugging techniques. If you need more personalized guidance, online programming assignment help services can offer tailored solutions.
7. Test with Different Inputs
Providing various test cases can help determine whether the program is handling all possible inputs correctly. Edge cases should also be considered to ensure robustness.
8. Use Version Control Systems
Using tools like Git allows you to track changes in your code, making it easier to revert to a previous version if needed. This can be helpful in identifying when an error was introduced.
9. Check for Infinite Loops and Recursion Issues
Loops and recursion can sometimes result in infinite execution, leading to crashes or timeouts. Make sure there are proper exit conditions in place.
10. Consult an Expert
If debugging becomes too challenging, seeking professional coding assignment help can save time and effort. Experts can provide guidance on best practices and optimize your code for better performance.
Debugging in Different Programming Languages
Debugging in Python
-
Use the built-in
pdb
module for step-by-step execution. -
Utilize
print()
statements to track variable values. -
Leverage IDEs like PyCharm for efficient debugging.
Debugging in Java
-
Use the Eclipse or IntelliJ IDEA debugger.
-
Check exception messages and stack traces.
-
Implement logging mechanisms with
Log4j
.
Debugging in C++
-
Use
gdb
for debugging in Unix-based systems. -
Check memory usage with tools like Valgrind.
-
Look out for segmentation faults and memory leaks.
Debugging in JavaScript
-
Use the browser’s Developer Console.
-
Implement
console.log()
for debugging. -
Utilize breakpoints in Chrome DevTools.
If you need further assistance, programming assignment help Australia services offer specialized support for debugging in various languages.
Preventing Errors in Coding Assignments
While debugging is essential, preventing errors from occurring in the first place can save significant time. The following are recommended procedures to adhere to:
-
Follow Coding Standards: Adhering to language-specific conventions improves readability and reduces errors.
-
Write Modular Code: Breaking the code into functions and modules makes debugging easier.
-
Comment Your Code: Adding meaningful comments helps in understanding the code better.
-
Use Proper Data Structures: Choosing the right data structures can enhance efficiency and reduce errors.
-
Perform Code Reviews: Having someone else review your code can provide a fresh perspective on potential issues.
-
Regular Testing: Implementing unit testing and test-driven development (TDD) ensures that your code is functioning correctly at every stage.
Conclusion
Debugging is an inevitable part of programming, but with the right techniques, it can become an easier and more structured process. Understanding common errors, using debugging tools, and following best practices can help you resolve issues efficiently. If you are struggling with debugging, seeking coding assignment help or Online Programming Assignment Help services can provide professional assistance to improve your code quality and understanding.
By mastering debugging techniques, you can enhance your problem-solving skills, boost your confidence, and excel in your programming assignments. Happy coding!
FAQs
What is debugging in coding?
Debugging is the process of identifying, analyzing, and fixing errors in a program to ensure it runs correctly and efficiently.
What are the common types of programming errors?
The common types of programming errors include syntax errors, logical errors, runtime errors, compilation errors, and semantic errors.
What are the best tools for debugging?
Popular debugging tools include IDE debuggers (like PyCharm, Eclipse, and VS Code), gdb
for C++, Chrome DevTools for JavaScript, and logging frameworks like Log4j
.
How can I avoid errors in my coding assignments?
To avoid errors, follow coding standards, write modular code, comment your code, perform code reviews, and use unit testing.
Where can I get help with debugging?
If you need assistance, coding assignment help services and online programming assignment help platforms can provide expert guidance and solutions.
Leave a Reply